Programmer's manual
1. Overview
Figure 1 shows the general idea of a host application
using the ABCp library.
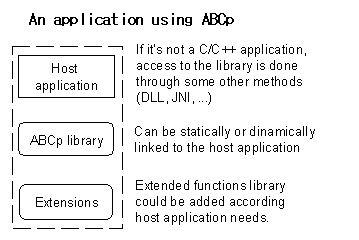
fig. 1
ABCp is an ANSI C library so it should be easy
to statically link it to the host application. All C/C++ compilers
will be able to do it and also many compiler for other languages
offer this possibility.
ABCp could also be compiled as a shared library
opening it up to other languages.
2. Interaction with the library
Figure 2 shows how the ABCp scanner reads the
abc file and translate it in a sequence of tokens that the host
program will handle.

fig.
2
The sequence diagram in figure 3 should clarify the way
the host application interacts with the library.
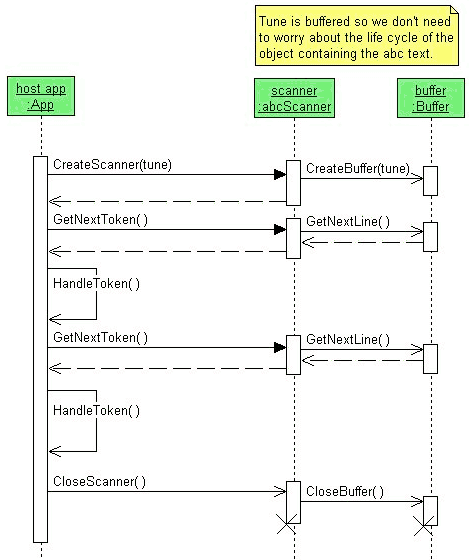
fig. 3
There is a rather important point to bear in mind. The ABCp
scanner only deals with "token", it does not check the
meaningfulness of the context in which they appear. For example
"A-B" is reported as:
"the note A, a tie, the note B". It's up to the host application to
decide if this is interpretable in any way or is to be
considered an error.
3. The scanner
As shown in the figure 3 above, the host application
interfaces the library through a scanner object. There are two
functions to create a scanner:
abcScanner *abcFileScanner(char
*filename,unsigned long bufsize);
abcScanner
*abcStringcanner(unsigned char *abctext, unsigned
long bufsize);
The first one reads the music from a file, the second one from a
text buffer.
4. An example
The following example shows a tipical usage of ABCp.
001: #include "abcp.h"
002: int main(int argc,char **argv)
003: {
004:
abcScanner *s; unsigned short tok;
005: s =
abcFileScanner(argv[1],0);
006:
if (s != NULL) {
007:
while ((tok =
abcNextToken(s)) != T_EOF)
008:
if (tok ==
T_NOTE)
009:
printf("Note:
%c%d\n",abcNote(s,pitch),abcNote(s,octave));
010:
}
011:
abcClose(s);
012: }
The code above, creates a scanner and get tokens one at a time
from the file whose name has been given as the first argument. For
each note, its pitch and octave are printed. The file main.c in the
distribution archive provide a complete example.
An effort has been made to keep an OO resemblance in the syntax.
Would the above example be in C++, one would have read:
s.NextToken() and
s.Note.pitch (or
something similar).
5. Tokens
The ABC file is read "token by token". The following functions
will help dealing with tokens:
unsigned
short abcNextToken(s) |
Reads a new token from the input and returns its
code. |
unsigned
short abcToken(s) |
Returns the code of the current token |
unsigned char
*abcTokenStart(s) |
The beginning of the string that matched the
token. Note that this is not a zero terminated string! |
unsigned short
abcTokenLength(s) |
The length of the matched string. |
Note that abcTokenStart(s) does not return a pointer to a zero
terminated string! If you are really interested in the string
itself you should copy it in a local buffer using, for example,
strncpy().
This is the list of the different tokens abcNextToken() may
return.
5.1 T_UNKNOWN
Returned when the scanner has been unable to recognize a piece
of input. It is normally a sign of a syntax error; for example:
A , B,
Will report the token T_UNKNOWN on the stray comma
after the note A.
This could also be used to enrich the ABC syntax. You could, for
instance, assign a meaning to '$' and being able to handle the
following:
A $ B
5.2 T_NONE
This token should never been reported by the scanner. In case
you get it , you can safely ignore it.
5.3 T_EOF
Marks the end of the ABC input. Any other call to abcNextToken()
will return T_EOF.
5.4 T_EMPTYLINE
A line made by 0 or more spaces (ASCII 32 or ASCII 9
characters) followed by a return. It's used to mark the end of an
ABC Tune.
5.5 T_ENDLINE
The end of a physical line. Normally marks the point where a
staff should be broken.
5.6 T_BREAK
An explicit request to break the line (!).
5.7 T_CONTINUE
An indication that the input line ends but the logical lines
actually continues (\). It may be followed by a comment (\ %...) in
which case the functions defined for T_COMMENT will return a
non-null value.
5.8 T_COMMENT
A comment (%....). The following functions will help
retrieving the comment string:
unsigned char
*abcComment(s,string) |
The beginning of the the comment string. Note
that this is not a zero terminated string! |
unsigned short
abcComment(s,length) |
The length of the comment string. |
5.9 T_PRAGMA
A line starting with '#'. This may be used by host applications
to introduce extended functions.
5.10 T_FIELD
An information field. The following functions will help with
fields.
5.10.1 General functions
char abcField(s,error) |
A code for possible errors/warnings. |
char abcField(s,field) |
The letter distinguish the field. |
5.10.2 String fields
The string that appears after the field specifier.
unsigned char
*abcField(s,string) |
The beginning of the string with the field
content |
unsigned short
abcField(s,length) |
The length of the above string |
This is can be used with any field and it's all that you need
for fields like T:, A:, O: etc.
5.10.3 L: Notes length
The length is specified as a fraction:
unsigned char
abcLength(s,num) |
Numerator |
unsigned char
abcLength(s,den) |
Denominator |
5.10.4 M: Meter
The meter is specified as a fraction (e.g. 4/4):
unsigned char
abcMeter(s,num) |
Numerator. |
unsigned char
abcMeter(s,den) |
Denominator. |
If abcMeter(s,num) returns 0, you can
check if it's a special meter using the following functions:
unsigned char
abcMeter(s,isCommon) |
Common time (M:C) |
unsigned char
abcMeter(s,isCut) |
Cut time (M:C|) |
unsigned char
abcMeter(s,isFree) |
Free time (M: free) |
If abcMeter(s,den) returns 1, it's an
ancient meter (M:3).
ABCp also accepts different meters for playing eg: M:3=6/4
unsigned char
abcMeter(s,num_play) |
Numerator to be used when playing |
unsigned char abcMeter(s,den_play) |
Denominator to be used when playing |
To retrieve addends of a complex meters (e.g. M:2+2+3/8) the following array is
available:
unsigned char abcMeter(s,num_adds)[] |
Up to ten addends for the numerator of a complex
meter. The first 0 value marks the end of addends.
(abcMeter(s,num_adds)[k]) |
The meter, its numerator or its denominator can be
parenthesized.
unsigned char abcMeter(s,brace) |
0: no parenthesis
3: denominator enclosed in parentesis (M:2/(4))
9: meter between parenthesis (M:(3/4))
12: numerator enclosed in parentesis (M:(3+2+2)/8)
15: both num and den enclosed in parentesis (M:(3)/(2))
All other values are due to unbalanced parenthesis and, thus,
meaningless. |
5.10.5 Q: Tempo
Tempo can be expressed in many ways. The one proposed in
the ABC 2.0 standard draft is Q:n/d=b to express that there are
b notes of length
n/d per minute.
The following functions help with this syntax:
unsigned char
abcTempo(s,num) |
Numerator
|
unsigned char abcTempo(s,den) |
Denominator
|
unsigned char abcTempo(s,beats) |
Beats per minute
|
Older ABC syntax allowed a tempo specification that was
dependent from the default note length: Q:C=120 or Q:120 means that
there are 120 notes of the default length in a minute. ABCp detects
it and set the isRelative field accordingly:
unsigned char
abcTempo(s,isRelative) |
True if tempo is relative to default note
length (e.g. Q:C3=40) |
For printing purpose up to four beats can be specified
(Q:1/4 3/8 1/4 3/8=40). The num and den fields will report the
correct sum (i.e. as if it was Q:5/4=40) and the numerators and
denominators to print will be in the nums and dens arrays.
unsigned char
abcTempo(s,nums)[] |
Numerators. The first 0 element marks the end of
values.
|
unsigned char abcTempo(s,dens)[] |
Denominators. The first 0 element marks the end
of values.
|
5.10.6 r: Remarks
See T_COMMENT.
5.10.7 K: Key
unsigned char
abcKey(s,tonic) |
The Key tonic:
A-G pitch
Z none
p hornpipe
P hornpipe (don't print key signature)
|
unsigned char abcKey(s,accidental) |
The key accidental
n none
s sharp
f flat
|
unsigned char abcKey(s,mode) |
Key mode:
M Major
N Minor
X Mixolydean
D Dorian
P Phrygean
Y Lydian
L Locridean
I Ionian
A Aeolian
E Explicit
|
unsigned char abcKey(s,signature)[] |
Explicit accidentals in the signature.
n none
N natural
s sharp
S double sharp
f flat
F double flat
Accidentals are stored according the ASCII order, not the
musical order (i.e. abcKey(s,signature)[0]
keeps accidentals for A).
|
The key field can also contain clef specifiers:
unsigned char
abcKey(s,clef) |
The clef :
N none
P percussion
C alto
F bass
G treble
d Doh (Gregorian)
f Fa (Gregorian)
T Tablature
|
unsigned char
abcKey(s,cleffline) |
The line where the clef is
to be placed.
|
unsigned char
abcKey(s,middle_pitch) |
The pitch of the note
standing on the middle line of the staff
|
unsigned char
abcKey(s,middle_octave) |
The octave of the note
standing on the middle line of the staff
|
Transposition specifiers:
char
abcKey(s,tr_octaves) |
The number of octaves to transpose (+/- 10)
|
char abcKey(s,tr_semitones) |
The number of semitones to transpose (+/-
120)
|
Staff parameters:
unsigned char
abcKey(s,stafflines) |
The number of lines in the
staff (0 .. 9)
|
unsigned char
abcKey(s,staves_grouped) |
The number of staves
(starting from the current one) connected to form a system.
|
unsigned char
abcKey(s,staves_grouping) |
The type of connection for
grouped staves:
| a vertical bar
[ a bracket
{ a brace
|
unsigned char
abcKey(s,space)
|
Defines or modifies the
vertical space between this staff and the one below it.
|
unsigned char
abcKey(s,stems)
unsigned char abcKey(s,gstems) |
To force direction of
notes (or grace notes) stems:
U up
D down
|
5.10.8 V: Voice
unsigned char
abcVoice(s,id_string) |
The beginning of the unique
voice identifier string
|
unsigned char
abcVoice(s,id_length) |
The length of the above
string
|
unsigned char
abcVoice(s,nm_string) |
The beginning of the voice
long name string (e.g. "Grand Piano")
|
unsigned char
abcVoice(s,nm_length) |
The length of the above
string
|
unsigned char
abcVoice(s,sn_string) |
The beginning of the voice
short name string (e.g. "G.P.")
|
unsigned char
abcVoice(s,sn_length) |
The length of the above
string
|
The Voice field can also contain clef specifiers:
unsigned char
abcVoice(s,clef) |
The clef :
N none
P percussion
C alto
F bass
G treble
d Doh (Gregorian)
f Fa (Gregorian)
T Tablature
|
unsigned char abcVoice(s,cleffline) |
The line where the clef is
to be placed.
|
unsigned char abcVoice(s,middle_pitch) |
The pitch of the note
standing on the middle line of the staff
|
unsigned char abcVoice(s,middle_octave) |
The octave of the note
standing on the middle line of the staff
|
Transposition specifiers:
char abcVoice(s,tr_octaves) |
The number of octaves to transpose (+/- 10)
|
char abcVoice(s,tr_semitones) |
The number of semitones to transpose (+/-
120)
|
Staff parameters:
unsigned
char abcVoice(s,stafflines) |
The number of lines in the
staff (0 .. 9)
|
unsigned
char abcVoice(s,staves_grouped) |
The number of staves
(starting from the current one) connected to form a system.
|
unsigned
char abcVoice(s,staves_grouping) |
The type of connection for
grouped staves:
| a vertical bar
[ a bracket
{ a brace
|
unsigned
char abcVoice(s,space)
|
Defines or modifies the
vertical space between this staff and the one below it.
|
unsigned
char abcVoice(s,stems)
unsigned char abcVoice(s,gstems) |
To force direction of
notes (or grace notes) stems:
U up
D down
|
5.10.9 P: Parts
TBD
5.11 T_NOTE
Returned each time a note is encountered.
unsigned
char abcNote(s,pitch) |
The note pitch, A-G
|
char abcNote(s,octave) |
The octave the note belongs to, -10 .. 10. 'C' in
ABCp is C4, 'c' is C5.
|
unsigned char abcNote(s,num)
unsigned char abcNote(s,den)
|
The length of the note expressed as a fraction of
the default length |
short abcNote(s,bend) |
Accidentals and microtones are treated as
bending the note pitch. A semitone is equivalent to a pitch
bend of 8192. The image below shows a progression by quarters
of tone (microtones notation is just one of the many used, there's
no standard!).
|

|
unsigned
char abcNote(s,bending) |
May have three
values:
\0 no bending
b bending
p cautionary (parentesized) bend (see image on the
right)
|
5.12 T_REST
unsigned
char abcRest(s,type) |
May have three values:
z rest
x invisible rest
Z multibar rest
|
unsigned char abcRest(s,num)
unsigned char abcRest(s,den)
|
The length of the rest expressed as a fraction of
the default length for z and x or as the number of bars
for Z.
|
5.13 T_SPACE
White space is used to separate notes that should not be
beamed. Black space (obtained with `) is used to make the suorce
ABC file more readable.
unsigned
char abcSpace(s,type) |
May have two values:
W White (space and tabs)
B Black (`)
|
unsigned char abcSpace(s,spaces)
|
The number of space characters.
|
5.14 T_SPACER
A spacer (y) is used to insert typographical white space in the
line.
unsigned
char abcSpacer(s,unit) |
May have four values:
m millimeters
c centimeters
i inches
p points
|
unsigned char abcSpace(s,space)
|
The amount of space to insert.
|
5.15 T_DECORATION
ABCp is able to recognize many commonly used decorations and
also allows synonyms (e.g. "pp" and "pianissimo" are considered the
same).
It returns a unique code for each:
unsigned char
*abcDecoration(s,string) |
The start of the decoration
string.
|
unsigned short
abcDecoration(s,length) |
The length of the above
string.
|
unsigned short
abcDecoration(s,code) |
If the decoration is not
recognized, the code is 0.
See following sections for codes.
|
5.15.1 Dynamic signs
Code
(hex) |
Example |
Decoration |
2101 |
|
pppp pianissississimo |
2102 |
|
ppp pianississimo |
2103 |
|
pp pianissimo |
2104 |
|
p piano |
2105 |
|
mp mezzopiano |
2106 |
|
mf mezzoforte |
2107 |
|
f forte |
2108 |
|
ff fortissimo |
2109 |
|
fff fortississimo |
210A |
|
ffff fortissississimo |
210B |
|
fp fortepiano |
210C |
|
sfz fz sforzando |
210D |
|
rfz rf rinforzando |
210E |
|
sfp sforzandopiano |
210F |
|
niente n |
2110 |
|
sffff subitoffff subitofortissississimo |
2111 |
|
sfff subitofff subitofortississimo |
2112 |
|
sff subitoff subitofortissimo |
2113 |
|
sf subitof subitoforte |
2114 |
|
smf subitomf subitomezzoforte |
2115 |
|
smp subitomp subitomezzopiano |
2116 |
|
sp subitop subitopiano |
2117 |
|
spp subitopp subitopianissimo |
2118 |
|
sppp subitoppp subitopianississimo |
2119 |
|
spppp subitopppp subitopianissississimo |
211C |
|
crescendo |
211D |
|
<( |
211E |
|
<) |
2120 |
|
diminuendo |
2121 |
|
>( |
2122 |
|
>) |
2125 |
|
glissando g |
5.15.2 Articulation
Code
(hex) |
Example |
Decoration |
2A01 |
|
accent emphasis > |
2A02 |
|
marcato |
2A03 |
|
staccatissimo |
2A04 |
|
staccato |
2A05 |
|
tenuto |
2A06 |
|
portato |
2A07 |
|
upbow |
2A08 |
|
downbow |
2A09 |
|
flageolet |
2A0A |
|
thumb |
2A0B |
|
lheel |
2A0C |
|
rheel |
2A0D |
|
ltoe |
2A0E |
|
rtoe |
2A0F |
|
open |
2A10 |
|
stopped |
2A12 |
|
reverseturn invertedturn |
2A13 |
|
trill tr |
2A14 |
|
prall |
2A15 |
|
mordent lowermordent |
2A16 |
|
prallprall |
2A17 |
|
prallmordent |
2A18 |
|
upprall |
2A19 |
|
downprall |
2A1A |
|
upmordent uppermordent pralltriller |
2A1B |
|
downmordent |
2A1C |
|
pralldown |
2A1D |
|
prallup |
2A1E |
|
lineprall |
2A1F |
|
signumcongruentiae |
2A20 |
|
slide |
2A22 |
|
reverseturnx invertedturnx |
2A25 |
|
roll irishroll ~ |
2A30 |
|
turn |
2A31 |
|
turnb |
2A32 |
|
bturn |
2A33 |
|
turn# |
2A34 |
|
#turn |
2A35 |
|
turnx |
2A36 |
|
xturn |
2A37 |
|
turnbb |
2A38 |
|
bbturn |
2A39 |
|
turn## |
2A3A |
|
##turn |
5.15.3 Fingering
Code
(hex) |
Example |
Decoration |
2201 |
|
1 |
2202 |
|
2 |
2203 |
|
3 |
2204 |
|
4 |
2205 |
|
5 |
2206 |
|
arpeggio |
2207 |
|
arpeggioup |
2208 |
|
arpeggiodown |
2209 |
|
arrow |
220A |
|
arrowup |
220B |
|
arrowdown |
220C |
|
noarpeggio |
220D |
|
H.O. hammeringon |
220E |
|
P.O. pulloff |
220F |
|
SL. sliding |
2210 |
|
W. wham |
2211 |
|
strum |
2212 |
|
strumup |
2213 |
|
strumdown |
2214 |
|
m.g. mg. lefthand |
2215 |
|
m.d. md. righthand |
2216 |
|
pizzicato |
2217 |
|
bartokpizzicato snappizzicato |
5.15.4 Pedals
Code
(hex) |
Example |
Decoration |
2401 |
|
damppedal ped |
2402 |
|
sostpedal mped |
2404 |
|
softpedal lped |
5.15.5 Pause
Code
(hex) |
Example |
Decoration |
2501 |
|
breath |
2502 |
|
fermata hold H |
2503 |
|
shortfermata |
2504 |
|
longfermata |
2505 |
|
verylongfermata |
2506 |
|
generalpause G.P. |
2507 |
|
caesura pause rr // |
2508 |
|
reversefermata invertedfermata ufermata udfermata |
2509 |
|
grandpause |
5.15.6 Percussion
Code
(hex) |
Example |
Decoration |
2301 |
|
Gong |
2302 |
|
Triangle |
2303 |
|
HalfOpenHiHat |
2304 |
|
HardStick |
2305 |
|
SoftStick |
2306 |
|
MetalStick |
2307 |
|
RubberStick |
2308 |
|
TriangleStick |
2309 |
|
WireBrush |
230A |
|
WoodStick |
2326 |
|
Snare |
232A |
|
HiHat |
5.15.7 Misc
Code
(hex) |
Example |
Decoration |
2601 |
|
segno |
2602 |
|
coda |
2603 |
|
D.S. |
2604 |
|
D.C. |
2605 |
|
dacoda |
2606 |
|
dacapo |
2607 |
|
dalsegno |
2608 |
|
fine |
2609 |
|
varcoda |
260A |
|
shortphrase |
260B |
|
mediumphrase |
260C |
|
longphrase |
260D |
|
8va |
260F |
|
8vab |
2611 |
|
beamon |
2612 |
|
gmark |
2613 |
|
invisible |
2620 |
|
shead |
2621 |
|
xhead |
2622 |
|
chead |
2623 |
|
dhead |
2624 |
|
sfhead |
2625 |
|
xfhead |
2626 |
|
cfhead |
2627 |
|
dfhead |
2640 |
|
stemup |
2641 |
|
stemdown |
5.15.8 Decoration range
Some decoration has a "range".
Examples might be pedal duration: "A +ped(+ B C D E +ped)+
F"
or temporary transposition: "... A | +8va(+ B C D
+8va)+ E | F ..."
To check if braces are present, use the following function:
unsigned char
abcDecoration(s,braces) |
May be:
0 No brace
1 Closing brace
2 Opening brace
|
5.16 T_BAR
unsigned
char abcBar(s,type) |
May have the
following values:
0 Non standard bar
1 |
2 ||
3 |]
4 [|
5 [ or ]
6 []
7 [|]
8 .|
|
unsigned char
*abcRepeat(s,string)
|
The start of the bar
string
|
unsigned short
abcRepeat(s,length)
|
The length of the above
string
|
unsigned short
abcRepeat(s,end_repeat)
|
The bar closes a section
that should be repeated n times.
|
unsigned short
abcRepeat(s,start_repeat)
|
The bar starts a section
that should be repeated n times.
|
5.17 T_TUPLET
unsigned
char abcTuplet(s,notes)
unsigned char abcTuplet(s,time)
unsigned char abcTuplet(s,span) |
In its general form a tuplet is notated with
three numbers as follows:
(n:t:s with the
meaning: 'put n notes into the time of t spanning next s notes'.
|
5.18 T_GCHORD
Each guitar chord is split into its elements:
unsigned char
abcGChord(s,rootnote)
|
The root note (e.g. C in
"Cmin")
|
unsigned char
abcGChord(s,rootaccidental) |
The accidental (e.g. 'b' in
"Db"). May be 'b' or '#'
|
unsigned char
abcGChord(s,bassnote)
|
The bass note (e.g. F in
"G/F")
|
unsigned char
abcGChord(s,bassaccidental)
|
The bass accidental (e.g.
'#' in "Dmin/E#")
|
unsigned char
*abcGChord(s,string)
|
The start of the chord
string
|
unsigned
short abcGChord(s,length)
|
The length of the above
string
|
unsigned
short abcGChord(s,type) |
The chord type (major,
minor, ...) according the following table:
01 |
(major) |
02 |
sus2 |
03 |
7sus2 |
04 |
dim |
05 |
m7b5 m7-5 |
06 |
dim7 |
07 |
- m min |
08 |
m7 |
09 |
m9 |
0A |
m11 |
0B |
m13 |
0C |
m711 |
0D |
m/maj7 mM7 mmaj7 m+7 |
0E |
m/maj9 mM9 mmaj9 m9+7 |
0F |
m6 |
10 |
add4 |
11 |
b5 -5 |
12 |
6-5add9 6b5add9 6-5/9 6b5/9 |
13 |
7-5 7b5 |
14 |
7-5-9 7b5b9 |
15 |
9b5 |
16 |
2 add2 |
17 |
7 |
18 |
7-9 7b9 dim9 |
19 |
9 |
1A |
11 |
1B |
13 |
1C |
7#9 7+9 |
1D |
M7 maj7 |
1E |
M9 maj9 |
1F |
maj11 |
20 |
maj13 |
21 |
add9 |
22 |
6 |
23 |
6/9 6add9 add6/9 |
24 |
#5 + aug +5 |
25 |
7+ 7#5 7+5 aug7 |
26 |
9+ 9#5 9+5 aug9 |
27 |
7+b9 7+-9 |
28 |
4 |
29 |
sus sus4 |
2A |
6sus4 |
2B |
6sus4add9 6sus4/9 |
2C |
7sus4 |
2D |
9sus4 |
2E |
M7sus4 maj7sus4 |
2F |
M9sus4 maj9sus4 |
30 |
no3rd 5 |
31 |
7sus9 |
32 |
add9no3rd sus9 |
Note that alternative names are supported. Also, spaces and
parenthesis are ignored so that A7(sus2) and A7sus2 denote the same
chord.
|
An auxiliary function helps with guitar chords. Each chord is
made by three or more notes played togheter, the
following function helps determine which notes are in a
chord
unsigned long
abcGChordNotes(int type,
unsigned char *steps) |
Returns a 32 bit integer
where bits are set on notes that belong to that chord. If steps is
not NULL, it is assumed to be an array of at least 10 unsigned char
and is filled with the notes that belong to the chord.
|
For example, abcGChordNotes(1,notes), will fill the notes array
with the values: 7,4,0 because the major chord contains the root
note, the 3rd (that is at 4 semitones from the root note) and the
5th (that is at 7 semitones from the root note).
Similarly, abcGChordNotes(8,notes) will fill notes with
10,7,3,0 because the 7th minor is made by the root note, the flat
3rd (3 semitones), the 5th (7 semitones) and the flat 7th (10
semitones).
5.19 T_CHORDSTART
Marks the start of a chord as in [CEG]. Chord notes will be reported one
at the time.
5.20 T_CHORDEND
Marks the start of a chord as in [CEG].
5.21 T_GRACEAPP
Marks the start of an appoggiatura as in {acg}. Grace notes will be reported one
at the time.
5.22 T_GRACEACC
Marks the start of an appoggiatura as in {/acg}. Grace notes will be
reported one at the time.
5.23 T_GRACEEND
Marks the end of grace notes as in {acg}.
5.24 T_SLURSTART
Marks the start of a slur as in (C D ^F).
unsigned char
abcSlur(s,direction) |
The slur direction:
\0 auto
U up
D down
|
unsigned char abcSlur(s,dotted) |
0 solid
1 dotted
|
5.25 T_SLUREND
Marks the start of a slur as in (C D ^F).
5.26 T_TIE
A tie as in C2-C.
unsigned char
abcTie(s,direction) |
The tie direction:
\0 auto
U up
D down
|
unsigned char abcTie(s,dotted) |
0 solid
1 dotted
|
5.27 T_ANNOTATION
unsigned char
abcAnnotation(s,position) |
Position relative to the
note the annotation is aligned with:
< left
> right
^ up
_ down
@ centered
|
unsigned char
abcAnnotation(s,x_offset) |
Offset in the x
direction
|
unsigned char
abcAnnotation(s,y_offset) |
Offset in the y
direction
|
unsigned char
*abcAnnotation(s,string)
|
The start of the annotation
string
|
unsigned
short abcGAnnotation(s,length)
|
The length of the above
string
|
5.28 T_INCLUDE
Signal the beginning of an inclusion.
unsigned char
*abcInclude(s,filename)
|
The name of the file that
is about to be included. Not a zero terminated string!
|
unsigned
short abcInclude(s,length)
|
The length of the above
string
|
You may want not to include that files (for example if you're
searching for a tune within a file). In that case you may use the
following function:
unsigned char
*abcStopInclude(s)
|
Prevent the inclusion of
the file.
|
5.29 T_INCLUDE_EOF
Signal the end of the included file.
5.30 T_LYR_SYLLABLE
A syllable in a w: field.
unsigned char
*abcLyrics(s,syllable) |
The start of the syllable
string.
|
unsigned
short abcLyrics(s,length)
|
The length of the above
string
|
5.31 T_LYR_BLANK
An empty syllable (*)
5.32 T_LYR_BAR
A bar occuring in a w: field.
5.33 T_LYR_SPACE
A space in a w: field.
unsigned short
abcLyrics(s,spaces) |
The number of space
characters.
|
5.34 T_LYR_HOLD
Holding the last syllable (_).
5.35 T_LYR_VERSE
unsigned short
abcLyrics(s,verse) |
The number of the
verse.
|
5.36 T_LYR_CONTINUE
A continuation happened in a w: field. Use function in T_COMMENT to check the existance of comments.
5.37 T_SYM_BLANK
An empty symbol (*).
5.38 T_SYM_BAR
A bar occuring in a s: field.
5.39 T_SYM_VERSE
unsigned short
abcDecoration(s,verse) |
The number of the
verse.
|
5.40 T_SYM_CONTINUE
A continuation happened in a s: field. Use function in T_COMMENT to check the existance of comments.
5.41 T_SYM_GCHORD
A guitar chord in a s: field. See T_GCHORD
for details.
5.42 T_SYM_DECORATION
A decoration in a s: field. See T_DECORATION for details.
5.43 T_SYM_ANNOTATION
An annotation in a s: field. See T_ANNOTATION for details.
5.44 T_BROKENRIGHT
For broken rhythms.
unsigned char
abcBroken(s,dots) |
The number of dots (eg.
C>>D).
|
5.45 T_BROKENLEFT
For broken rhythms.
unsigned char
abcBroken(s,dots) |
The number of dots (eg.
C<<D).
|
5.46 T_XFIELD
Extended field (%%...).
unsigned char
*abcXField(s,key_string) |
The start of field key
string (e.g. MIDI in %%MIDI).
|
unsigned short
abcXField(s,key_length) |
The length of the above
string
|
unsigned char
*abcXField(s,val_string) |
The start of field value
string (e.g. A4 in %%papersize A4).
|
unsigned short
abcXField(s,val_length) |
The length of the above
string
|
unsigned short
abcXField(s,key) |
The numeric code for the
key (or 0 if key is not recognized):
1881 |
papersize |
1882 |
propagate-accidentals |
1883 |
score staves |
1884 |
writeout-accidentals |
1885 |
deco |
1886 |
postscript |
1901 |
barnumbers |
1902 |
barsperstaff |
1903 |
measurefirst |
1904 |
measurenb |
1905 |
setbarnb |
1A01 |
lineskipfac |
1A02 |
maxshrink |
1A03 |
parskipfac |
1A04 |
sep |
1A05 |
scale |
1B01 |
continueall |
1B02 |
exprabove |
1B03 |
exprbelow |
1B04 |
freegchord |
1B05 |
musiconly |
1B06 |
measurebox |
1B07 |
graceslurs |
1B08 |
infoline |
1B09 |
landscape |
1B0A |
oneperpage |
1B0B |
printtempo |
1B0C |
stretchlast |
1B0D |
stretchstaff |
1B0E |
vocalabove |
1B0F |
withxrefs |
1B10 |
writehistory |
1C01 |
botmargin |
1C02 |
composerspace |
1C03 |
indent |
1C04 |
infospace |
1C06 |
leftmargin |
1C07 |
musicspace |
1C08 |
pageheight |
1C09 |
pagewidth |
1C0A |
partsspace |
1C0B |
rightmargin |
1C0C |
staffsep |
1C0D |
subtitlespace |
1C0E |
sysstaffsep |
1C0F |
textspace |
1C10 |
titlespace |
1C11 |
topmargin |
1C12 |
topspace |
1C13 |
vocalspace |
1C14 |
wordsspace |
1D01 |
annotationfont |
1D02 |
composerfont |
1D03 |
gchordfont |
1D04 |
infofont |
1D06 |
partsfont |
1D07 |
subtitlefont |
1D08 |
setfont-1 |
1D09 |
setfont-2 |
1D0A |
setfont-3 |
1D0B |
setfont-4 |
1D0C |
tempofont |
1D0D |
textfont |
1D0E |
titlefont |
1D0F |
vocalfont |
1D10 |
wordsfont |
1E01 |
center |
1E02 |
text |
1E03 |
abc-copyright |
1E04 |
abc-version |
1E05 |
abc-creator |
1E06 |
abc-charset abc-encoding |
1E07 |
abc-include include |
1E08 |
abc-edited-by |
1F01 |
begintext textbegin |
1F02 |
endtext textend |
1F03 |
newpage |
1F04 |
vskip |
|
5.47 T_OVERLAY
For creating layers within a voice (&).
5.48 T_OVERLAYSTART
For creating layers within a voice ( &( ).
5.49 T_OVERLAYEND
For creating layers within a voice ( &) ).
Example: | A B &( C D & E F &) |
5.50 T_DOVERLAY
For creating layers within a voice (&&).
5.51 T_DOVERLAYSTART
For creating layers within a voice ( &&( ).
5.52 T_DOVERLAYEND
For creating layers within a voice ( &&) ).
6. Builiding ABCp library
6.1 Makefile
TBW
6.2 Strings
ABCp is able to recognise and return unique codes for many
strings used in the ABC syntax. In some case the usefulness of this
has been challenged.
For example, if you are using ABCp to create MIDI files,
you are not interested in instructions like topmargin or setfont-1,
having those string included in the library is nothing but a waste
of space.
The strings.c file contain a mechanism to exclude sets of
strings. If this is the case you can add -- EXCLUDE :PRINT: at the
beginning of strings.c and those strings won't be included in the
library.
6.3 yrx
To ease the creation of ABCp, I wrote a tool to match
regular expression based on the public domain regular
expression package by Yigit
Ozan.
You can find it in the tools directory.
|